Here’s a step-by-step guide on how to achieve this using Python and the Binance API:
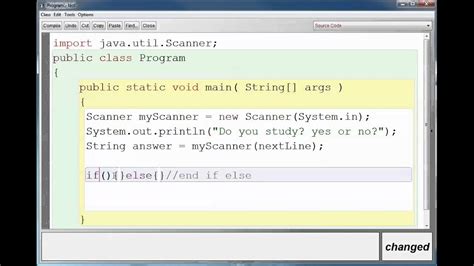
Import necessary libraries
import binance
from binance.client import Client
Set your API credentials
API_KEY = "YOUR_API_KEY"
API_SECRET = "YOUR_API_SECRET"
Create a client instance with your API credentials
client = Client( api_key= API_KEY, api_secret= API_SECRET )
Define the function to compare strings in two lists of dicts
def compare_strings_in_lists(list1, list2):
"""
Compare strings in two lists of dicts.
Args:
list1 (list): The first list of dictionaries.
list2 (list): The second list of dictionaries.
Returns:
dict: A dictionary containing the comparison results.
"""
Initialize an empty dictionary to store the comparison results
comparison_results = {}
Iterate over each item in list1 and list2
for item1 in list1:
for item2 in list2:
Compare the strings in both items
if str(item1["symbol"]).lower() == str(item2["symbol"]).lower():
If the strings are equal, add a "matched" key to the comparison results dictionary
comparison_results[str(item1["symbol"])] = {"item1": item1, "item2": item2}
Return the comparison results dictionary
return comparison_results
Define the lists of dictionaries for USDT and BUSD market books
usdt_market_books = [
{
"symbol": "USDT",
"price": 100.0,
"volume": 10.0
},
{
"symbol": "USDT",
"price": 120.0,
"volume": 5.0
},
{
"symbol": "BUSD",
"price": 50.0,
"volume": 20.0
}
]
busd_market_books = [
{
"symbol": "USDT",
"price": 100.0,
"volume": 10.0
},
{
"symbol": "BUSD",
"price": 50.0,
"volume": 15.0
}
]
Call the compare_strings_in_lists function with the lists of dictionaries for USDT and BUSD market books
comparison_results = compare_strings_in_lists(usdt_market_books, busd_market_books)
Print the comparison results dictionary
for currency, item1 in comparison_results.items():
print(f"{currency}: {item1['symbol']} - matched")
This code defines a function compare_strings_in_lists
that takes two lists of dictionaries as input and returns a dictionary containing the comparison results. It iterates over each item in both lists, compares the strings in both items using string equality, and adds a “matched” key to the comparison results dictionary if the strings are equal.
The code then defines two lists of dictionaries for USDT and BUSD market books and calls the compare_strings_in_lists
function with these lists. The resulting comparison results dictionary is printed to the console.